Increased speed and flexibility are important for the Agile team in automated testing (AT). In addition, tests must be understandable even for non-technical people. These criteria play a special role in choosing tools for daily QA testers’ work. In this tutorial, we will discuss Codeception — the modern PHP testing framework, which QA engineers value for its usability and extensive capabilities.
What is Codeception?
Codeception is a popular framework designed for testing PHP web applications. it provides an absolutely new way of writing unit, functional, integration, and acceptance tests in a single, coherent style. Inspired by BDD, all Codeception tests are written in a descriptive manner.
Despite the fact that it is based on PHP, the user needs only basic knowledge for starting work with the framework, thanks to the set of custom commands offered by Codeception
Codeception works with 20+ modules, including Selenium, Laravel, Symfony, Yii, Phalcon, Joomla, etc. The module defines the actions available to you when writing tests. It also allows you to integrate with libraries with minimal customization and avoid the need to install additional plugins.
The Codeception framework is an open-source project with an MIT license.
Automation Testing With Codeception
Codeception is multifunctional because it allows QAs to comprehensively check web applications for compliance with business requirements and the absence of technical flaws thanks to implemented BDD approach.
You will require below pre-requisites:
- PHP (latest recommended) Check with the official PHP site by running the command
php -v php --version
- Composer
composer require "codeception/codeception" --dev
First Codeception Automated tests
And as we mentioned at the top Codeception uses three types of tests. Module and functional tests require access to the project’s PHP files, but you can do without Webserver. Acceptance tests require drivers for Firefox/Chrome and Webserver. ↪️ Let’s look at their features with examples:
#1: Acceptance tests
Page in browser acts as the scope of the acceptance test, e.g., Chrome, Firefox, PhpBrowser; JavaScript is supported. The Codecept framework makes the tests clean and readable. Let’s say your first test is log in. You will need a basic knowledge of PHP and HTML to write it.
You will get such a scenario in the acceptance test:
$I->amOnPage('/login');
$I->fillField('username', 'davert');
$I->fillField('password', 'qwerty');
$I->click('LOGIN');
$I->see('Welcome, Davert!');
You can use PhpBrowser or WebDriver to run this acceptance test. The first option is the easiest and fastest because the engine is Guzzle and Symfony BrowserKit. However, it does not support JS. In the second case, you need a full-fledged browser, Chrome or Firefox, working in headless mode.
#2: Functional tests
Behavior is similar to the previous view, but the test is executed inside PHP, requiring no web server and no JavaScript. You can use Symfony BrowserKit to “send” requests to the product under test.
Note that the syntax is similar to the previous test:
namespace Tests\Functional;
use \Tests\Support\FunctionalTester;
class LoginCest
{
public function tryLogin(FunctionalTester $I)
{
$I->amOnPage('/');
$I->click('Login');
$I->fillField('Username', 'Miles');
$I->fillField('Password', 'Davis');
$I->click('Enter');
$I->see('Hello, Miles', 'h1');
// $I->seeEmailIsSent(); // only for Symfony
}
}
Functionality tests are faster than acceptance tests but less stable. The reason is that the application and the framework run in the same environment.
#3: Unit tests & Integration tests
Such Codeception tests allow you to ensure the functions work correctly and, as a result, provide stable code.
PHPUnit is used as the backend, so you can add any test to the test suite and execute it afterwards. To do this, apply the following command:
- Create test through generate command: test and parameters (suite and test names):
php vendor/bin/codecept generate:test unit Example
- Run new test using the following command:
php vendor/bin/codecept run unit ExampleTest
- Run the whole set of tests:
php vendor/bin/codecept run unit
Test created with generate:test:
namespace Tests\Unit;
use \Tests\Support\UnitTester;
class ExampleTest extends \Codeception\Test\Unit
{
protected UnitTester $tester;
protected function _before()
{
}
// tests
public function testMe()
{
}
}
When it comes to a separate PHP class (unit test):
namespace Tests\Unit;
use \Tests\Support\UnitTester;
class UserTest extends \Codeception\Test\Unit
{
public function testValidation()
{
$user = new \App\User();
$user->setName(null);
$this->assertFalse($user->validate(['username']));
$user->setName('toolooooongnaaaaaaameeee');
$this->assertFalse($user->validate(['username']));
$user->setName('davert');
$this->assertTrue($user->validate(['username']));
}
}
When it comes to the integration test with modules (they are available here in the same way as in the previous two cases):
# Codeception Test Suite Configuration
# suite for unit (internal) tests.
actor: UnitTester
modules:
enabled:
- Asserts
- Db
So, one framework can replace several testing tools for your tasks. Using it, you will write all tests in a single style, ensuring consistency and transparency of the process. No wonder many Agile teams choose it as the basis for their project work.
Why Do Many QA Teams Choose Codeception?
QA engineers choose this framework for many reasons. We have highlighted 5 main advantages of test automation with Codeception that are especially valuable for everyday use:
- Multiframe and multi-backend. The framework allows you to work within the engine of your choice. A single syntax is used for all backends.
- Code clarity. You create the test as simply as possible in the form of a script. In essence, it repeats the actions of the app user, who clicks on links, fills out forms, waiting to see the result.
- Modularity. The built-in modules allow you to execute tests in different environments and for different purposes. You can use helper functions by creating a custom module “Helper” in the tests directory. You can also access other modules.
- Data cleanup. Thanks to the Db test database module, the database is regularly cleaned. Thus, the database is reset before each test, guaranteeing data consistency and preventing failures.
- Parallel run. Multithreading reduces test execution time. It is also an opportunity to check the correctness of code, which should work in multi-threaded mode.
Given the extensive capabilities of the tool, we decided to provide access to it for all Testomat.io users.
Sync Codeception Tests With Manual Testing
You can use our test management system to choose Codeception as the main framework for your project. It is available to you without restrictions, along with other options: Cypress.io, Playwright, Webdriber.io, Cucumber, Jasmine, etc.
Integrations are made via Build-In Importer and do not require complex configuration. Automated and manual tests are instantly synchronized. Thus, you get the ability to manage them in a single space.
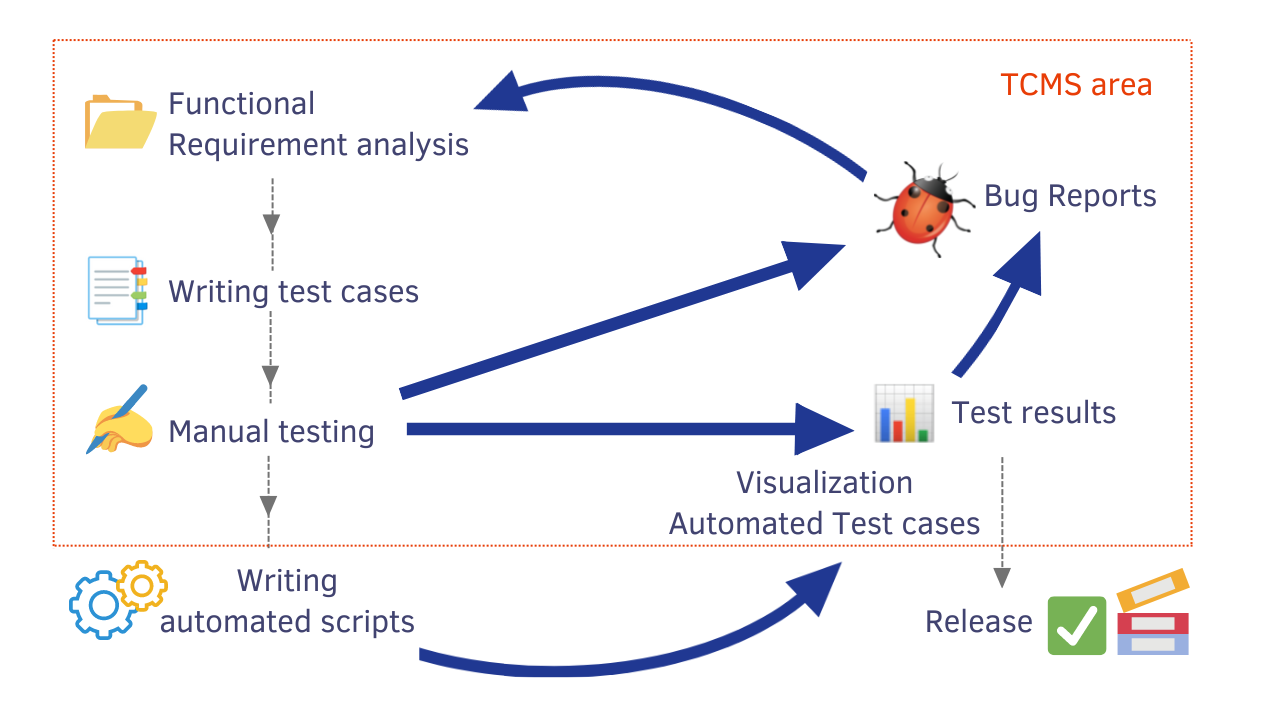
Want to get started with the framework in an agile TMS? Below we will tell you how to do it ⬇️
Getting Started Codeception From Example
Our team prepared a bunch of Demo projects for success starting with the testomat.io TCMS. Besides WebdriverIO following through the link, you can find Cypress, Playwright, Cucumber, Jest, CodeceptJS and other examples of testing frameworks. Download the needed project there and try how it works by yourself.
A team consisting of testers and specialists without QA (BA, PM) experience can work in our TMS. If necessary, the product owner is also involved in the project. You just need to take a few simple steps to provide a foundation for teamwork.
Steps you need to follow to run the example on testomat.io:
#1: Create A New Test Project
After registering in the system (or logging into your account), click Create project. Then come up with a Title that corresponds to the essence of your project. The Repository URL line is optional. Finally, click Create button.
#2: Import Codeception Automated Tests Into TMS
Here, you need to install Codeception from the list of frameworks. In this case, only PHP is available as a language. Then decide on OS: Mac, Linux, or Windows.
A special command and an API key will be generated for you. Copy this command and execute it in CMD. This starts the import of tests from the source code into the TMS. After then login to your project to open tests and explore them in more detail. Organize your Codeception tests in the best way.
TMS allows you to structure and manage test cases in a few clicks:
- create new test cases and delete unnecessary ones
- change the sequence
- move test cases between test suites
- filter by tags and priorities for quick retrieval
TMS automatically tracks changes in added tests. If the current version differs from the one imported from the source code, the system assigns an Out of Sync status.
You can not only import tests into a project but also write them manually. To write a test, select Create Suite from the menu and then Add new test. An Editor opens in front of you, where you can title the test using tags and formatting, insert links, tables, and buffer attachments, and add steps and snippets. When everything is ready, click Save.
The Project Timeline or Pulse feature is available to track changes within a project. You can see what has been changed, by whom, and when, and make decisions about rollback or restoration of the previous version.
How Do I Create A Report In Codeception?
You can view test results using an advanced reporter, which must be integrated with Codeception.
#3 Install Reporter
To do this, you must install Reporter in the repository by copying and adding a special command into the command line.
#4 Execute Codeception Scripts
When all steps have been completed, you should run the test.
TESTOMATIO_URL=https://beta.testomat.io TESTOMATIO='API' php vendor/bin/codecept run --ext "Testomatio\Reporter\Codeception"
#5 Review test result report and analytics
You will have real-time access to the results as you run it.
Track your progress and study the details of each test case (code, attachments, etc.). You can also access the results of manual tests.
Thus, you can view all the data associated with automated and manual tests in a single interface thanks to reports and in-depth analytics.
Our system has advanced analytical capabilities. The analytics are based on the detailed reports we discussed above.
Click the Analytics tab to see up-to-date information about automation coverage, failures, tests (ever-failing, slowest, flakiest, never run), and environments. Here you can also filter data by environment (Windows, macOS, Android, iOS, etc.) and tags.
Access to data about each test and suite can be used to make decisions about the project not only by the QA engineer but also by other team members.
TMS supports integrations with all popular CI/CD tools:
- Jenkins
- Bamboo
- GitHub
- GitLab
- Circle CI
- Azure Pipelines
You can speed up the product release process thanks to shorter feedback loops and the automation of routine tasks. This way, the Agile team can test quickly, covering all aspects of the product and identifying bugs early on.
Comparison table Codeception vs. CodeceptJS vs. Playwright
Codeception | CodeceptJS | Playwright |
PHP framework for unit, functional, and acceptance tests | JS-based E2E test framework | JS-based E2E tool for web apps |
Supports PHP | Supports JavaScript | Supports JS, Python, Java, C# |
Parallel testing | Parallel run with run-workers and run-multiple engines | Parallel tests |
Actively supports | Actively supports | Actively supports |
Firefox, Chrome, Safari, Cloud Testing with Selenium WebDriver | Google Chrome, Microsoft Edge, Firefox, Safari, Electron; Android, iOS | Chromium, WebKit, Firefox, Google Chrome for Android and Mobile Safari; Windows, Linux, macOS |
Provides an absolutely new way of writing tests, inspired by BDD | Uses special BDD-style syntax from the user’s point of view | Has Codegen function for generating test cases in the programming language of your choice |
Codeception is a decent option for an Agile workflow because it provides flexibility in writing, using, and maintaining tests. Moreover, it is realistic to perform different kinds of testing with a single framework.
By integrating Codeception with testomat.io, you create an optimal test environment for all team members, regardless of their technical expertise.